In this Project we will make “Bomb Puzzle Game” with Arduino. The “Bomb Puzzle Game” is an interactive game designed using Arduino Uno, a 4×4 keypad, a YX5300 MP3 module, and a TM1637 seven-segment display. The game consists of two sequential puzzles which user have to complete within predefined time limit:
- Cable Puzzle: The player must insert wires into two holes with the correct configuration. If successful, a success sound is played, and the game progresses to the next puzzle.
- Password Puzzle: The player must enter a predefined password using the keypad within a set time limit. If successful, a positive sound is played; otherwise, a “blast” sound is triggered.
Success triggers a positive sound, while failure results in a “Bomb Blast” sound effect, enhancing the game’s realism and excitement.
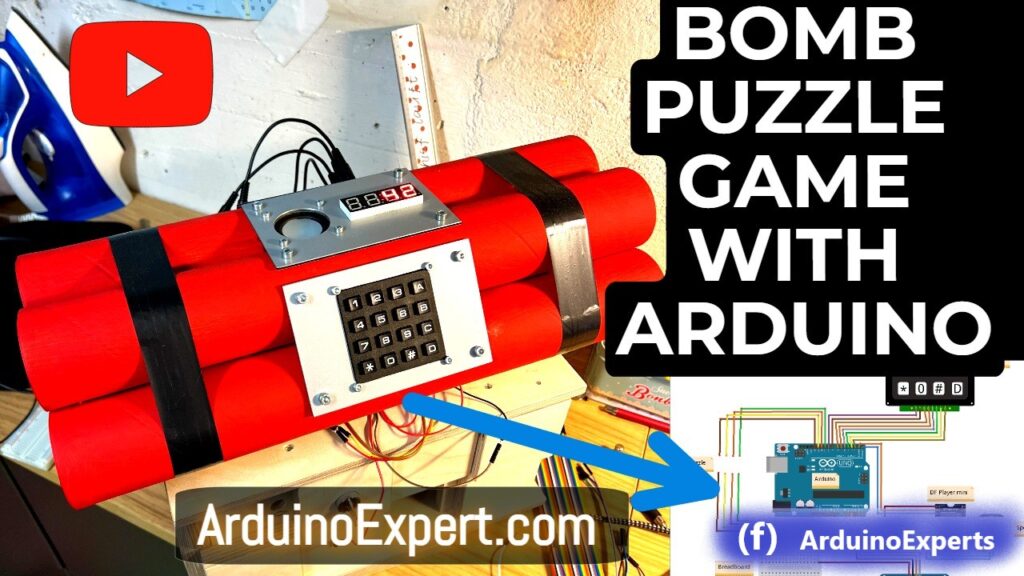
Components used in Bomb Puzzle Game:
- Arduino Uno: The microcontroller to control the game logic.
- 4×4 Keypad: Used for user input to enter the password.
- YX5300 MP3 Module: Plays sound effects (e.g., success, failure and blast sounds).
- TM1637 Seven-Segment Display: Displays the countdown timer.
- Cable Puzzle Mechanism: Two input holes and wires for the first puzzle.
- Speaker: Connected to the MP3 module for audio output.
- Power Supply: To power the Arduino and peripherals.
- Connecting Wires: For interfacing the components.
- Resistors and Breadboard: For prototyping connections.
Project Work Flow:
- Game Initialization:
- The system initializes when powered on.
- The TM1637 seven-segment display shows the countdown timer.
- The YX5300 MP3 module plays a “game start” sound.
- Cable Puzzle:
- The player inserts wires into two designated holes.
- The Arduino verifies the configuration using digital input pins.
- If the configuration is correct, a success sound is played, and the game progresses to the password puzzle.
- If incorrect, the player can retry until successful.
- Password Puzzle:
- The player enters a predefined password using the 4×4 keypad.
- On Each keypress Tick sound is played.
- Countdown Timer:
- The timer counts down from a predefined time (e.g., 60 seconds).
- The TM1637 display updates every second.
- Password Validation:
- If the correct password is entered within the time limit:
- The timer stops.
- The YX5300 MP3 module plays a success sound (e.g., “Success or defused”).
- The game resets for another round.
- If the time limit is exceeded:
- The YX5300 MP3 module plays a blast sound.
- The game resets for another round.
- If the correct password is entered within the time limit:
Circuit Diagram:
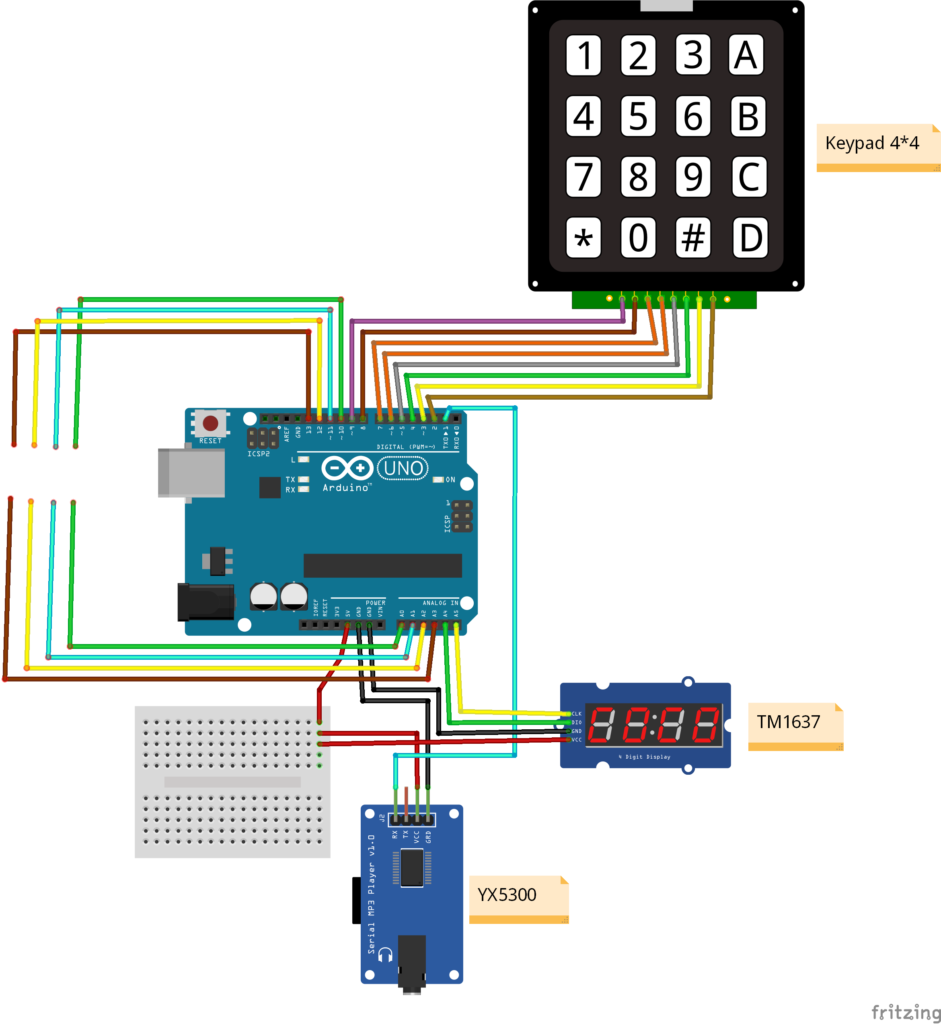
Code:
#include <Keypad.h>
//#include <SerialMP3Player.h>
#include "Adafruit_LEDBackpack.h"
//#include <TM1637Display.h>
#include "Arduino.h"
#include "DFRobotDFPlayerMini.h"
DFRobotDFPlayerMini myDFPlayer;
// MP3 player------------------------------------------
//SerialMP3Player mp3(0,1);
// Display---------------------------------------------
Adafruit_7segment matrix = Adafruit_7segment();
// Display---------------------------------------------
//#define DIO_PIN A4 // DIO (Data)
//#define CLK_PIN A5 // CLK (Clock)
//TM1637Display display(CLK_PIN, DIO_PIN);
int totalTime = 1800; // Timer value in seconds
int minutes, seconds;
unsigned long prevmls, prevmls1, prevmls2;
// Keypad Puzzle---------------------------------------
const byte ROW_NUM = 4;
const byte COL_NUM = 4;
char keys[ROW_NUM][COL_NUM] = {
{'1', '2', '3', 'A'},
{'4', '5', '6', 'B'},
{'7', '8', '9', 'C'},
{'*', '0', '#', 'D'}
};
byte rowPins[ROW_NUM] = {9, 8, 7, 6};
byte colPins[COL_NUM] = {5, 4, 3, 2};
Keypad keypad = Keypad(makeKeymap(keys), rowPins, colPins, ROW_NUM, COL_NUM);
// Correct Code for Puzzle
String correctCode = "0303#";
String enteredCode = "";
int ind = 0;
bool K_Puzzle = false, Game_Over = false;
bool flag=false;
// Cable Puzzle----------------------------------------
const int Left_pins[4] = {A0, A1, A2, A3};
const int Right_pins[4] = {10, 11, 12, 13};
int correctCableOrder[4] = {0, 1, 2, 3};
bool C_Puzzle;
int count = 0;
int CONEC[4] = {0, 0, 0, 0};
//------------------------------------------------------
void setup() {
// Initialize MP3 player
//mp3.begin(9600);
//delay(500); // wait for init
//mp3.sendCommand(CMD_SEL_DEV, 0, 2); //select sd-card
//delay(500);
// Set volume (0 to 30)
//mp3.setVol(30);
//mp3.play(05); //5 Play track Game Started
Serial.begin(9600); // Initialize Serial pins 0 and 1 for communication
delay(2000);
if (!myDFPlayer.begin(Serial)) { // Use Serial to communicate with mp3
while (true) {
delay(0); // Prevent watchdog reset
}
}
Project Demo Video:
Conclusion:
The “Bomb Puzzle Game” project provides a captivating and educational experience by combining multiple hardware components and programming logic. Players engage in two sequential challenges that test their problem-solving skills and attention to detail. The modular design allows for easy customization and expansion, making it an excellent choice for hobbyists and educators alike.
Need This Project?:
If you need this Project with or without Modifications or Customization then you can contact us through WhatsApp. We can deliver you this Project in the Following Ways.
- Fully Functional Project with Hardware/Components Shipment:
if you can not make this project yourself then you can use this option. We will assemble the Project and will ship it to your Doorstep with Safe Packaging. - Project Code:
we can provide you Project Code along with Zoom Assistant, through Zoom meeting for Setup of this Project or any other Arduino Project of your need.